Openpyxl Cheat Sheet
- A Workbook may have as less as one sheet and as many as dozens of worksheets. Active sheet is the worksheet user is viewing or viewed before closing the file. Each sheet consists of vertical columns, known as Column starting from A. Each sheet consists of rows, called as Row. Numbering starts from 1. Row and column meet at a box called Cell.
- $ pip install openpyxl After you install the package, you should be able to create a super simple spreadsheet with the following code: from openpyxl import Workbook workbook = Workbook sheet = workbook.active sheet'A1' = 'hello' sheet'B1' = 'world!'
- Openpyxl is a library in Python with which one can perform the different mathematic operations in an excel sheet. In this tutorial, we are going to learn the implementation of mathematical functions available in openpyxl library. Mathematical operations like.
- From openpyxltemplates.tablesheet import TableSheet from openpyxltemplates.tablesheet.columns import CharColumn, IntColumn class DemoTableSheet (TableSheet): column1 = CharColumn column2 = IntColumn The column declaration supports inheritance, the following declaration is perfectly legal.
- Openpyxl Cheat Sheet Free
- Openpyxl Cheat Sheet Printable
- Openpyxl Cheat Sheet Template
- Openpyxl Sheet By Name
Python openpyxl module is a perfect choice to work with excel sheets. We can also add images to the excel sheet by using the pillow library with it. But, it doesn’t guard us against quadratic blowup or billion laughs XML attacks.
Create a workbook¶
There is no need to create a file on the filesystem to get started with openpyxl.Just import the Workbook
class and start work:
A workbook is always created with at least one worksheet. You can get it byusing the Workbook.active
property:
Note
This is set to 0 by default. Unless you modify its value, you will alwaysget the first worksheet by using this method.

You can create new worksheets using the Workbook.create_sheet()
method:
Sheets are given a name automatically when they are created.They are numbered in sequence (Sheet, Sheet1, Sheet2, …).You can change this name at any time with the Worksheet.title
property:
The background color of the tab holding this title is white by default.You can change this providing an RRGGBB
color code to theWorksheet.sheet_properties.tabColor
attribute:
Once you gave a worksheet a name, you can get it as a key of the workbook:
You can review the names of all worksheets of the workbook with theWorkbook.sheetname
attribute
You can loop through worksheets
You can create copies of worksheets within a single workbook:
Workbook.copy_worksheet()
method:
Note
Only cells (including values, styles, hyperlinks and comments) andcertain worksheet attribues (including dimensions, format andproperties) are copied. All other workbook / worksheet attributesare not copied - e.g. Images, Charts.
You also cannot copy worksheets between workbooks. You cannot copya worksheet if the workbook is open in read-only or write-onlymode.
Playing with data¶
Accessing one cell¶
Now we know how to get a worksheet, we can start modifying cells content.Cells can be accessed directly as keys of the worksheet:
This will return the cell at A4, or create one if it does not exist yet.Values can be directly assigned:
There is also the Worksheet.cell()
method.
This provides access to cells using row and column notation:
Note
When a worksheet is created in memory, it contains no cells. They arecreated when first accessed.
Warning
Because of this feature, scrolling through cells instead of accessing themdirectly will create them all in memory, even if you don’t assign them a value.
Something like
will create 100x100 cells in memory, for nothing.
Accessing many cells¶
Ranges of cells can be accessed using slicing:
Ranges of rows or columns can be obtained similarly:
You can also use the Worksheet.iter_rows()
method:
Likewise the Worksheet.iter_cols()
method will return columns:
Note
For performance reasons the Worksheet.iter_cols()
method is not available in read-only mode.
If you need to iterate through all the rows or columns of a file, you can instead use theWorksheet.rows
property:
or the Worksheet.columns
property:
Note
For performance reasons the Worksheet.columns
property is not available in read-only mode.
Values only¶
If you just want the values from a worksheet you can use the Worksheet.values
property.This iterates over all the rows in a worksheet but returns just the cell values:
Both Worksheet.iter_rows()
and Worksheet.iter_cols()
cantake the values_only
parameter to return just the cell’s value:
Data storage¶
Once we have a Cell
, we can assign it a value:
Saving to a file¶
The simplest and safest way to save a workbook is by using theWorkbook.save()
method of the Workbook
object:
Warning
This operation will overwrite existing files without warning.
Note
The filename extension is not forced to be xlsx or xlsm, although you might havesome trouble opening it directly with another application if you don’tuse an official extension.
As OOXML files are basically ZIP files, you can also open it with yourfavourite ZIP archive manager.
Saving as a stream¶
If you want to save the file to a stream, e.g. when using a web applicationsuch as Pyramid, Flask or Django then you can simply provide aNamedTemporaryFile()
:
You can specify the attribute template=True, to save a workbookas a template:
or set this attribute to False (default), to save as a document:
Warning
You should monitor the data attributes and document extensionsfor saving documents in the document templates and vice versa,otherwise the result table engine can not open the document.
Note
The following will fail:
Loading from a file¶
The same way as writing, you can use the openpyxl.load_workbook()
toopen an existing workbook:
This ends the tutorial for now, you can proceed to the Simple usage section
Worksheet is the 2nd-level container in Excel.
openpyxl.worksheet.worksheet.
Worksheet
(parent, title=None)[source]¶Bases: openpyxl.workbook.child._WorkbookChild
Represents a worksheet.
Do not create worksheets yourself,use openpyxl.workbook.Workbook.create_sheet()
instead
BREAK_COLUMN
= 2¶
BREAK_NONE
= 0¶
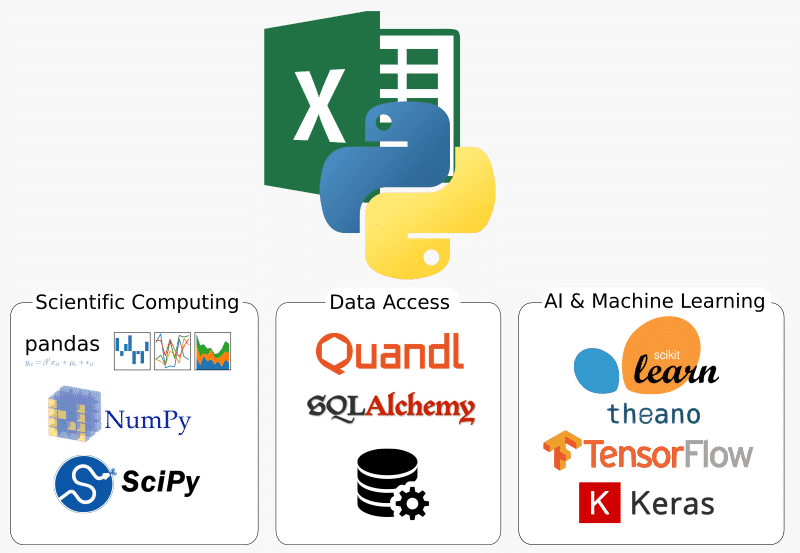
BREAK_ROW
= 1¶
ORIENTATION_LANDSCAPE
= 'landscape'¶
ORIENTATION_PORTRAIT
= 'portrait'¶
PAPERSIZE_A3
= '8'¶
PAPERSIZE_A4
= '9'¶
PAPERSIZE_A4_SMALL
= '10'¶
PAPERSIZE_A5
= '11'¶
PAPERSIZE_EXECUTIVE
= '7'¶
PAPERSIZE_LEDGER
= '4'¶
PAPERSIZE_LEGAL
= '5'¶
PAPERSIZE_LETTER
= '1'¶
PAPERSIZE_LETTER_SMALL
= '2'¶
PAPERSIZE_STATEMENT
= '6'¶
PAPERSIZE_TABLOID
= '3'¶
SHEETSTATE_HIDDEN
= 'hidden'¶
SHEETSTATE_VERYHIDDEN
= 'veryHidden'¶
SHEETSTATE_VISIBLE
= 'visible'¶
active_cell
¶
add_chart
(chart, anchor=None)[source]¶Add a chart to the sheetOptionally provide a cell for the top-left anchor
add_data_validation
(data_validation)[source]¶Add a rules='none'>Parameters:iterable (list|tuple|range|generator or dict) – list, range or generator, or dict containing values to append
Usage:
- append([‘This is A1’, ‘This is B1’, ‘This is C1’])
- or append({‘A’ : ‘This is A1’, ‘C’ : ‘This is C1’})
- or append({1 : ‘This is A1’, 3 : ‘This is C1’})
Raise: | TypeError when iterable is neither a list/tuple nor a dict |
---|
calculate_dimension
()[source]¶Return the minimum bounding range for all cells containing data (ex. ‘A1:M24’)
Return type: | string |
---|
cell
(row, column, value=None)[source]¶Returns a cell object based on the given coordinates.
Usage: cell(row=15, column=1, value=5)
Calling cell creates cells in memory when theyare first accessed.
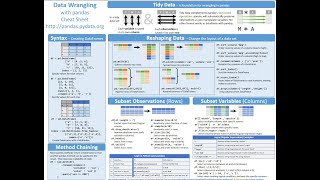
Parameters: |
|
---|---|
Return type: |
columns
¶Produces all cells in the worksheet, by column (see iter_cols()
)
delete_cols
(idx, amount=1)[source]¶Delete column or columns from colidx
delete_rows
(idx, amount=1)[source]¶Delete row or rows from rowidx
dimensions
¶Returns the result of calculate_dimension()
freeze_panes
¶
insert_cols
(idx, amount=1)[source]¶Insert column or columns before colidx
insert_rows
(idx, amount=1)[source]¶Insert row or rows before rowidx
iter_cols
(min_col=None, max_col=None, min_row=None, max_row=None, values_only=False)[source]¶Produces cells from the worksheet, by column. Specify the iteration rangeusing indices of rows and columns.
If no indices are specified the range starts at A1.
If no cells are in the worksheet an empty tuple will be returned.
Parameters: |
|
---|---|
Return type: | generator |
iter_rows
(min_row=None, max_row=None, min_col=None, max_col=None, values_only=False)[source]¶Produces cells from the worksheet, by row. Specify the iteration rangeusing indices of rows and columns.
If no indices are specified the range starts at A1.
If no cells are in the worksheet an empty tuple will be returned.
Parameters: |
|
---|---|
Return type: | generator |
max_column
¶The maximum column index containing data (1-based)
Type: | int |
---|
max_row
¶The maximum row index containing data (1-based)
Type: | int |
---|
merge_cells
(range_string=None, start_row=None, start_column=None, end_row=None, end_column=None)[source]¶Set merge on a cell range. Range is a cell range (e.g. A1:E1)
merged_cell_ranges
¶Return a copy of cell ranges
mime_type
= 'application/vnd.openxmlformats-officedocument.spreadsheetml.worksheet+xml'¶
min_column
¶The minimum column index containing data (1-based)
Type: | int |
---|
min_row
¶The minimium row index containing data (1-based)
Type: | int |
---|
move_range
(cell_range, rows=0, cols=0, translate=False)[source]¶Move a cell range by the number of rows and/or columns:down if rows > 0 and up if rows < 0right if cols > 0 and left if cols < 0Existing cells will be overwritten.Formulae and references will not be updated.
page_breaks
¶
print_area
¶The print area for the worksheet, or None if not set. To set, supply a rangelike ‘A1:D4’ or a list of ranges.
print_title_cols
¶Openpyxl Cheat Sheet Free
Columns to be printed at the left side of every page (ex: ‘A:C’)
print_title_rows
¶Rows to be printed at the top of every page (ex: ‘1:3’)
print_titles
¶
Openpyxl Cheat Sheet Printable
rows
¶Produces all cells in the worksheet, by row (see iter_rows()
)
Type: | generator |
---|
selected_cell
¶
set_printer_settings
(paper_size, orientation)[source]¶Set printer settings
sheet_view
¶
show_gridlines
¶
show_summary_below
¶
show_summary_right
¶
Openpyxl Cheat Sheet Template
tables
¶
unmerge_cells
(range_string=None, start_row=None, start_column=None, end_row=None, end_column=None)[source]¶Remove merge on a cell range. Range is a cell range (e.g. A1:E1)
values
¶Produces all cell values in the worksheet, by row
Type: | generator |
---|
Openpyxl Sheet By Name
openpyxl.worksheet.worksheet.
isgenerator
(obj)¶
